This procedure documents an automated method to globally update all users’ Microsoft 365 Entra Business Phone number fields from an M365 Teams Phone assigned phone number. if a phone number is assigned to the user. If the user has an assigned Microsoft 365 Teams Phone number, the function will replace the user’s business phone field with the registered phone number. The field will only be updated if different from the users assigned Microsoft 365 Teams Phone number. Users without an assigned Microsoft 365 Teams Phone number will not be modified.
The number field will be properly formatted in a common US format for phone numbers: (xxx) xxx-xxxx.
Next updates: I will be looking at setting this up as an Azure Automation to update periodically.
Some interesting, related notes:
- Updating users with users with privileged administrator roles requires you to have additional rights.
- The Business Phone field is considered sensitive user property. See What are sensitive actions.
- You cannot use API permissions; you must use Delegated. See Who can perform sensitive actions.
Prerequisites
- You must have Microsoft Graph PowerShell permissions to update the user’s Entra record: User.ReadWrite.All, Directory.AccessAsUser.All
- You must have these modules installed:
- Microsoft.Graph
- MicrosoftTeams
Install Modules
Install-Module -Name Microsoft.Graph -Scope CurrentUser
Install-Module -Name MicrosoftTeams -Scope CurrentUser
Connect to Graph and Teams
If you do not have the required rights, you will be prompted to add them. Do not enable Admin Consent, User Consent is adequate.
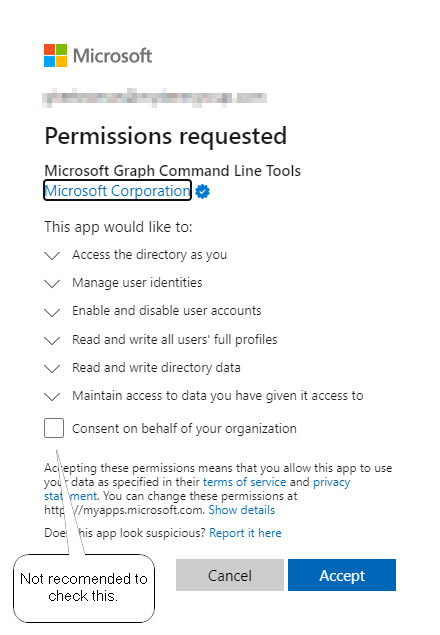
# Connect to MGGraph
Connect-MgGraph -Scopes "User.ReadWrite.All, Directory.AccessAsUser.All" -NoWelcome
# Connect MicrosoftTeams
Connect-MicrosoftTeams
Load the helper functions
These two functions will perform the actions along with error checking and properly format phone numbers.
<#
.SYNOPSIS
Updates a user's office phone number in Microsoft 365 if it is not already up to date.
.DESCRIPTION
This function updates the office phone number of a user in Microsoft 365 based on the provided UserPrincipalName and LineUri.
It includes error checking and cleans up the LineUri to a proper format by removing "tel:" if present. The function only updates the phone number if it is not already set to the provided value.
.PARAMETER UserPrincipalName
The UserPrincipalName (email address) of the user whose office phone number needs to be updated.
.PARAMETER LineUri
The phone number to be set as the office phone number. This should be in E.164 format.
.EXAMPLE
Set-UserOfficePhone -UserPrincipalName "jdoe@example.com" -LineUri "tel:+1234567890"
.NOTES
Author: Your Name
Date: 2024-11-22
See permissions and Sensitive Actions: https://learn.microsoft.com/en-us/graph/api/resources/users?view=graph-rest-1.0
#>
function Set-UserOfficePhone {
[CmdletBinding()]
param (
[Parameter(Mandatory = $true)]
[ValidateNotNullOrEmpty()]
[string]$UserPrincipalName,
[Parameter(Mandatory = $true)]
[ValidateNotNullOrEmpty()]
[string]$LineUri,
[Parameter(Mandatory = $false)]
[switch]$VerboseOutput
)
try {
if ($VerboseOutput){
Write-Output "Processing user: $UserPrincipalName to $LineUri"
}
# Clean up the LineUri by removing "tel:" if present
$CleanLineUri = $LineUri -replace "^tel:", ""
# Validate the cleaned LineUri format
if ($CleanLineUri -notmatch "^\+\d+$") {
throw "Invalid LineUri format. It should be in E.164 format (e.g., +1234567890)."
}
$PhoneFormatted = Format-PhoneNumber -PhoneNumber $CleanLineUri
# Get the user from Microsoft 365
$user = Get-MgUser -Filter "userPrincipalName eq '$UserPrincipalName'"
if ($null -eq $user) {
throw "User with UserPrincipalName '$UserPrincipalName' not found."
}
# Check if the office phone number is already up to date
# BusinessPhones is referred as Office Phone in the M365 Admin Portal and Business phone in Entra.
$currentPhone = $user.BusinessPhones[0]
if ($currentPhone -eq $PhoneFormatted) {
Write-Output "The office phone number for user '$UserPrincipalName' is already up to date."
}
else {
# Update the user's office phone number
if ($VerboseOutput){
Write-Output "Calling: Update-MgUser -UserId $($user.Id) -BusinessPhones @($PhoneFormatted)"
}
Update-MgUser -UserId $user.Id -BusinessPhones @($PhoneFormatted)
Write-Output "Successfully updated office phone number for user '$UserPrincipalName' to '$PhoneFormatted'."
}
}
catch {
Write-Error "An error occurred: $_"
}
}
<#
.SYNOPSIS
Formats a phone number to the format +1(XXX) XXX-XXXX.
.DESCRIPTION
This function takes a phone number in various formats and converts it to the format +1(XXX) XXX-XXXX.
.PARAMETER PhoneNumber
The phone number to be formatted. It should contain at least 10 digits.
.EXAMPLE
Format-PhoneNumber -PhoneNumber "1234567890"
Output: +1(123) 456-7890
.NOTES
Author: Your Name
Date: 2024-11-22
#>
function Format-PhoneNumber {
[CmdletBinding()]
param (
[Parameter(Mandatory = $true)]
[ValidateNotNullOrEmpty()]
[string]$PhoneNumber
)
try {
# Remove any non-digit characters
$digitsOnly = $PhoneNumber -replace "\D", ""
# Ensure the phone number has at least 10 digits
if ($digitsOnly.Length -lt 10) {
throw "Phone number must contain at least 10 digits."
}
# Extract the last 10 digits for formatting
$lastTenDigits = $digitsOnly.Substring($digitsOnly.Length - 10)
# Format the phone number
$formattedNumber = "+1({0}) {1}-{2}" -f $lastTenDigits.Substring(0, 3), $lastTenDigits.Substring(3, 3), $lastTenDigits.Substring(6, 4)
return $formattedNumber
}
catch {
Write-Error "An error occurred: $_"
}
}
Run the code
This code will update the user’s Entra Business Phone field with the user’s assigned Microsoft Team’s Phone number. It will not change or remove phone numbers from a user’s record if there is no assigned Microsoft Team’s Phone number, as it is impossible to know if this was a manually entered or previously updated team’s phone.
# Get all Teams users with an assigned phone (LineUri)
$csUsers = get-csonlineuser -Filter {LineURI -ne $null -and AccountType -eq 'User' -and AccountEnabled -eq $true} | select Identity,UserPrincipalName,LineUri
# Update the user's business phone number
foreach ($user in $csUsers) {
Set-UserOfficePhone -UserPrincipalName $user.UserPrincipalName -LineUri $user.LineUri
}
Reference
Microsoft 365 Graph Update user function reference
Microsoft Entra built-in roles
Are you interested in more articles? Check out: Windows 10 End of Life: What It Means for Your Business and How to Upgrade to Windows 11